Electron APIs Misuse: An Attacker’s First Choice
16 Feb 2021 - Posted by Luca Carettoni, Lorenzo StellaElectronJs is getting more secure every day. Context isolation and other security settings are planned to become enabled by default with the upcoming release of Electron 12 stable, seemingly ending the somewhat deserved reputation of a systemically insecure framework.
Seeing such significant and tangible progress makes us proud. Over the past years we’ve committed to helping developers securing their applications by researching different attack surfaces:
- Modern Alchemy: Turning XSS into RCE
- Electron Windows Protocol Handler MITM/RCE (bypass for CVE-2018-1000006 fix)
- Subverting Electron Apps via Insecure Preload
- Signature Validation Bypass Leading to RCE In Electron-Updater
As confirmed by the Electron development team in the v11 stable release, they plan to release new major versions of Electron (including new versions of Chromium, Node, and V8), approximately quarterly. Such an ambitious versioning schedule will also increase the number and the frequency of newly introduced APIs, planned breaking changes, and consequent security nuances in upcoming versions. While new functionalities are certainly desirable, new framework’s APIs may also expose powerful interfaces to OS features, which may be more or less inadvertently enabled by developers falling for the syntactic sugar provided by Electron.
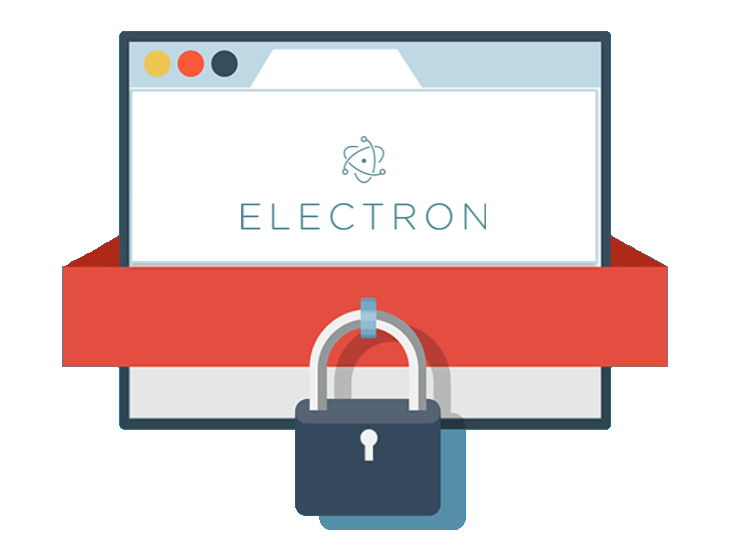
Such interfaces may be exposed to the renderer’s, either through preloads or insecure configurations, and can be abused by an attacker beyond their original purpose. An infamous example of this is openExternal
.
Shell’s openExternal()
allows opening a given external protocol URI with the desktop’s native utilities. For instance, on macOS, this function is similar to the open terminal command utility and will open the specific application based on the URI and filetype association. When openExternal is used with untrusted content, it can be leveraged to execute arbitrary commands, as demonstrated by the following example:
const {shell} = require('electron')
shell.openExternal('file:///System/Applications/Calculator.app')
Similarly, shell.openPath(path)
can be used to open the given file in the desktop’s default manner.
From an attacker’s perspective, Electron-specific APIs are very often the easiest path to gain remote code execution, read or write access to the host’s filesystem, or leak sensitive user’s data. Malicious JavaScript running in the renderer can often subvert the application using such primitives.
With this in mind, we gathered a non-comprehensive list of APIs we successfully abused during our past engagements. When exposed to the user in the renderer, these APIs can significantly affect the security posture of Electron-based applications and facilitate nodeIntegration / sandbox bypasses.
Remote.app
The remote module provides a way for the renderer processes to access APIs normally only available in the main process. In Electron, GUI-related modules (such as dialog, menu, etc.) are only available in the main process, not in the renderer process. In order to use them from the renderer process, the remote
module is necessary to send inter-process messages to the main process.
While this seems pretty useful, this API has been a source of performance and security troubles for quite a while. As a result of that, the remote
module will be deprecated in Electron 12, and eventually removed in Electron 14.
Despite the warnings and numerous articles on the topic, we have seen a few applications exposing Remote.app
to the renderer. The app
object controls the full application’s event lifecycle and it is basically the heart of every Electron-based application.
Many of the functions exposed by this object can be easily abused, including but not limited to:
app.relaunch([options])
Relaunches the app when current instance exits.app.setAppLogsPath([path])
Sets or creates a directory your app’s logs which can then be manipulated withapp.getPath()
orapp.setPath(pathName, newPath)
.app.setAsDefaultProtocolClient(protocol[, path, args])
Sets the current executable as the default handler for a specified protocol.app.setUserTasks(tasks)
Adds tasks to the Tasks category of the Jump List (Windows only).app.importCertificate(options, callback)
Imports the certificate in pkcs12 format into the platform certificate store (Linux only).app.moveToApplicationsFolder([options])
Move the application to the default Application folder (Mac only).app.setJumpList(categories)
Sets or removes a custom Jump List for the application (Windows only).app.setLoginItemSettings(settings)
Sets executables to launch at login with their options (Mac, Windows only).
Taking the first function as a way of example, app.relaunch([options])
can be used to relaunch the app when the current instance exits. Using this primitive, it is possible to specify a set of options, including a execPath
property that will be executed for relaunch instead of the current app along with a custom args
array that will be passed as command-line arguments. This functionality can be easily leveraged by an attacker to execute arbitrary commands.
Native.app.relaunch({args: [], execPath: "/System/Applications/Calculator.app/Contents/MacOS/Calculator"});
Native.app.exit()
Note that the relaunch method alone does not quit the app when executed, and it is also necessary to call app.quit()
or app.exit()
after calling the method to make the app restart.
systemPreferences
Another frequently exported module is systemPreferences. This API is used to get the system preferences and emit system events, and can therefore be abused to leak multiple pieces of information on the user’s behavior and their operating system activity and usage patterns. The metadata subtracted through the module could be then abused to mount targeted attacks.
subscribeNotification, subscribeWorkspaceNotification
These methods could be used to subscribe to native notifications of macOS. Under the hood, this API subscribes to NSDistributedNotificationCenter
. Before macOS Catalina, it was possible to register a global listener and receive all distributed notifications by invoking the CFNotificationCenterAddObserver
function with nil
for the name
parameter (corresponding to the event parameter of subscribeNotification
). The callback specified would be invoked anytime a distributed notification is broadcasted by any app. Following the release of macOS Catalina or Big Sur, in the case of sandboxed applications it is still possible to globally sniff distributed notifications by registering to receive any notification by name. As a result, many sensitive events can be sniffed, including but not limited to:
- Screen locks/unlocks
- Screen saver start/stop
- Bluetooth activity/HID Devices
- Volume (USB, etc) mount/unmount
- Network activity
- User file downloads
- Newly Installed Applications
- Opened Source Code Files
- Applications in Use
- Loaded Kernel Extensions
- …and more from the installed application including sensitive information in them. Distributed notifications will always be public by design, and it was never correct to put sensitive information in them.
The latest NSDistributedNotificationCenter
API also seems to be having intermittent problems with Big Sur and sandboxed application, so we expected to see more breaking changes in the future.
getUserDefault, setUserDefault
The getUserDefault
function returns the value of key in NSUserDefaults
, a macOS simple storage class that provides a programmatic interface for interacting with the defaults system. This systemPreferences
method can be abused to return the Application’s or Global’s Preferences. An attacker may abuse the API to retrieve sensitive information including the user’s location and filesystem resources. As a matter of demonstration, getUserDefault
can be used to obtain personal details of the targeted application user:
- User’s most recent locations on the file system
> Native.systemPreferences.getUserDefault("NSNavRecentPlaces","array") (5) ["/tmp/secretfile", "/tmp/SecretResearch", "~/Desktop/Cellar/NSA_files", "/tmp/blog.doyensec.com/_posts", "~/Desktop/Invoices"]
- User’s selected geographic location
Native.systemPreferences.getUserDefault("com.apple.TimeZonePref.Last_Selected_City","array") (10) ["48.40311", "11.74905", "0", "Europe/Berlin", "DE", "Freising", "Germany", "Freising", "Germany", "DEPRECATED IN 10.6"]
Complementarily, the setUserDefault
method can be weaponized to set User’s Default for the Application Preferences related to the target application. Before Electron v8.3.0 [1], [2] these methods can only get or set NSUserDefaults
keys in the standard suite.
Shell.showItemInFolder
A subtle example of a potentially dangerous native Electron primitive is shell.showItemInFolder
. As the name suggests, this API shows the given file in a file manager.
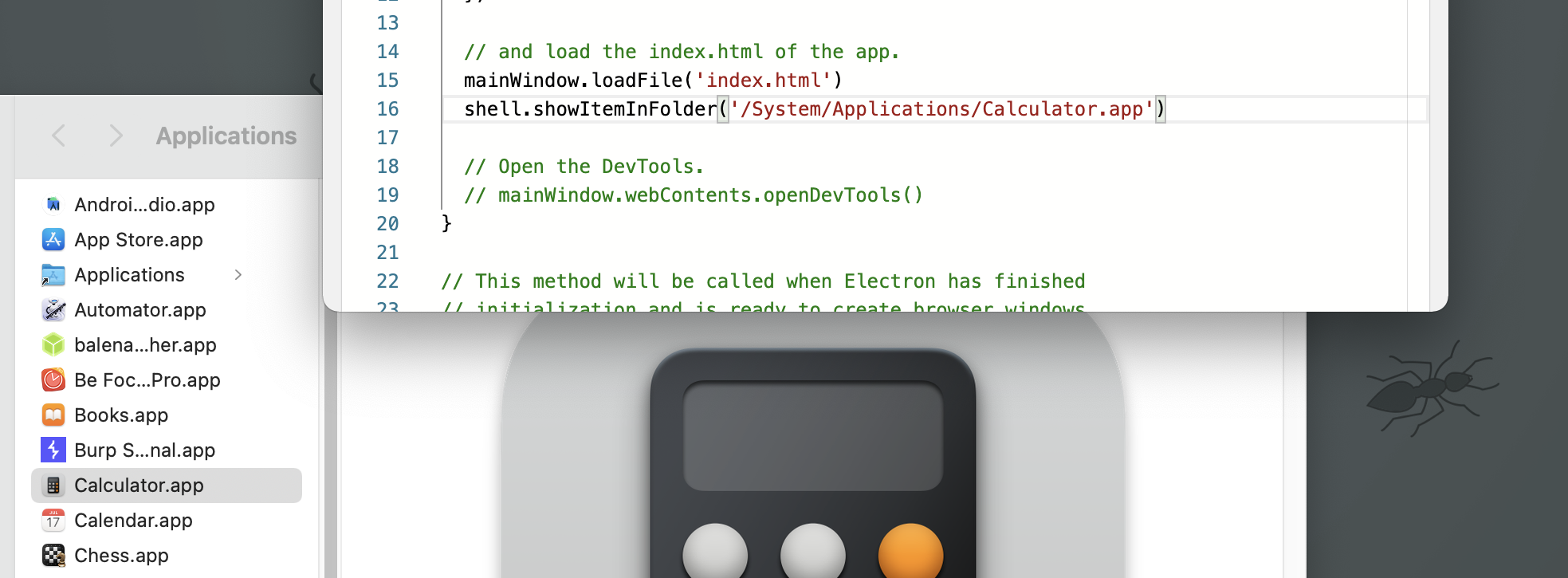
Such seemingly innocuous functionality hides some peculiarities that could be dangerous from a security perspective.
On Linux (/shell/common/platform_util_linux.cc
), Electron extracts the parent directory name, checks if the resulting path is actually a directory and then uses XDGOpen (xdg-open
) to show the file in its location:
void ShowItemInFolder(const base::FilePath& full_path) {
base::FilePath dir = full_path.DirName();
if (!base::DirectoryExists(dir))
return;
XDGOpen(dir.value(), false, platform_util::OpenCallback());
}
xdg-open
can be leveraged for executing applications on the victim’s computer.
“If a file is provided the file will be opened in the preferred application for files of that type” (https://linux.die.net/man/1/xdg-open)
Because of the inherited time of check time of use (TOCTOU) condition caused by the time difference between the directory existence check and its launch with xdg-open
, an attacker could run an executable of choice by replacing the folder path with an arbitrary file, winning the race introduced by the check. While this issue is rather tricky to be exploited in the context of an insecure Electron’s renderer, it is certainly a potential step in a more complex vulnerabilities chain.
On Windows (/shell/common/platform_util_win.cc
), the situation is even more tricky:
void ShowItemInFolderOnWorkerThread(const base::FilePath& full_path) {
...
base::win::ScopedCoMem<ITEMIDLIST> dir_item;
hr = desktop->ParseDisplayName(NULL, NULL,
const_cast<wchar_t*>(dir.value().c_str()),
NULL, &dir_item, NULL);
const ITEMIDLIST* highlight[] = {file_item};
hr = SHOpenFolderAndSelectItems(dir_item, base::size(highlight), highlight,
NULL);
...
if (FAILED(hr)) {
if (hr == ERROR_FILE_NOT_FOUND) {
ShellExecute(NULL, L"open", dir.value().c_str(), NULL, NULL, SW_SHOW);
} else {
LOG(WARNING) << " " << __func__ << "(): Can't open full_path = \""
<< full_path.value() << "\""
<< " hr = " << logging::SystemErrorCodeToString(hr);
}
}
}
Under normal circustances, the SHOpenFolderAndSelectItems
Windows API (from shlobj_core.h) is used. However, Electron introduced a fall-back mechanism as the call mysteriously fails with a “file not found” exception on old Windows systems. In these cases, ShellExecute
is used as a fallback, specifying “open” as the lpVerb
parameter. According to the Windows Shell documentation, the “open” object verb launches the specified file or application. If this file is not an executable file, its associated application is launched.
While the exploitability of these quirks is up to discussions, these examples showcase how innoucous APIs might introduce OS-dependent security risks. In fact, Chromium has refactored the code in question to avoid the use of xdg-open
altogether and leverage dbus
instead.
The Electron APIs illustrated in this blog post are just a few notable examples of potentially dangerous primitives that are available in the framework. As Electron will become more and more integrated with all supported operating systems, we expect this list to increase over time. As we often repeat, know your framework (and its limitations) and adopt defense in depth mechanisms to mitigate such deficiencies.
As a company, we will continue to devote our 25% research time to secure the ElectronJS ecosystem and improve Electronegativity.